For-Loops in Python
In all of the Python programs we've seen so far, the computer executes the commands one line at a time, from top to bottom. However, most programming languages contain several powerful features that can change the flow of the program. These statements that alter the flow of a program are called control flow statements. One such control flow statement is the ability to iterate--to repeat parts of the code.
Python uses a for-loop to repeat the same block of code a set number of times. Below is a for-loop that repeats three print statements five times (and then prints Bye
exactly once):
Data Science DISCOVERY Data Science DISCOVERY Data Science DISCOVERY Data Science DISCOVERY Data Science DISCOVERY Bye
There are a few features to notice:
- The line of code with the
for
statement ends in a colon (:
). This colon indicates that the next block of code should be repeated a number of times. - The number of times the for-loop repeats in indicated in what appears after the
in
statement. In the code above,range(5)
runs the code inside of the for-loop five times. - The block of code following the
for
statement is indented. This indentation defines the block of code that should run multiple times and we refer to this code as being "inside" of the for-loop. - The code after the for-loop, back at the original indentation, will only run after the for-loop finishes running.
Application of for-loops: Simulation
A simple and widely used application is to re-run a simulation multiple times and these for-loops nearly always use for i in range(17)
(where 17
is replaced with the number of times our simulation runs).
range(17)
is shorthand for listing out a long sequence of numbers starting with zero and going to one less than the end of the range --[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16]
-- that will be "looped through" by the for-loop.- The variable
i
will take on each of these each time it goes through the loop. Even if we never usei
, it's still required syntax in our for-loop.
Example: Printing i
in a for-loop
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
Ten cards randomly drawn (with replacement) using a for-loop.
Simulation Example: Repeating Rolling a Die
When we discussed generating Random Numbers in Python, we explored generating 100 rolls of a six-sided die. Now we can do this in just two lines of code:
1 4 3 6 6 3 3 3 5 3 5 4 6 2 4 3 2 5 4 1 6 3 4 1 3 2 4 1 6 6 3 6 2 4 2 3 3 1 3 1 3 3 5 2 4 2 3 5 5 5 5 2 4 1 1 1 2 6 6 1 6 3 5 4 1 2 5 1 4 1 5 5 3 3 2 4 4 5 2 5 2 6 3 6 5 2 5 2 5 1 6 5 3 3 2 5 5 4 2 2
One hundred randomly generated numbers using random.radint(1, 6)
, using a for-loop.
Simulation Example: Drawing 10 Cards (with replacement)
5 of Hearts 2 of Diamonds A of Spades 3 of Spades J of Clubs 8 of Spades 2 of Hearts 10 of Diamonds A of Clubs K of Clubs
Ten cards randomly drawn (with replacement) using a for-loop.
Application of for-loops: Iteration
The other major common application of a for-loop is to iterate through a list. This can be done using a Python list, such as the lists we use in random.choice
. Instead of using i
, the variable used between for
and in
should be descriptive of the data that it will contain. If we are looping through a list of suits of cards, the variable suit
makes a lot of sense.
Iteration Example: Python List of Card Suits
Club Heart Diamond Spade
Using a for-loop to visit every element in a Python List.
Iteration Example: Python List of Primary and Secondary Colors
Red Yellow Blue Orange Green Violet
Using a for-loop to visit every element in a Python List.
Example Walk-Throughs with Worksheets
Video 1: Examples of "for" Loops in Python
Practice Questions
Q1: Looking at the snippet of code below, how many times will the for loop execute?
Q2: Looking at the image below, which snippet of code will incorrectly print the list `data`?
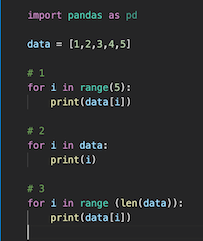