Functions in Python
A major component of being able to manipulate and analyze data in Python is being able to effectivetly use functions. Functions allow you to simplify repetitve processes or common tasks that you will encounter often when working with data. Below are a few functions that you've already been introduced to that are built into Pandas library in Python:
- df.max(): Returns the maximum value in each object.
- df.sum(): Returns the sum of values of an object.
- df.nlargest(): Selects and orders the top n entries.
The next step of using functions in Python is to be able to write your own functions for more specific uses. We will walk through the basic syntax for defining your own function by deconstructing the function below:
# Define a function to simulate rolling a six-sided die
def rollDie():
value = random.randint(1, 6)
return value
- In the first line of code for writing our function, we defined our function using def + (function name) + "()" + ":". All of these componenets need to be included when first defining a function.
- In the second line of code, we included what are function was actually doing. In this case we assigned a variable "value" to a random value between 1 and 6 to simulate the outcome of rolling a die one time. You can see that the line is indented, which will be the case for all lines of code included in a function after the initial line for defining the function.
- In the third line of code, we return the value we want our function to spit out when a user runs the function. The syntax of this will always be return + (variable we want to return). Notice that this line of code is indented as well.
Now let's take a look at what the function will return when run:
# Run rollDie()
roll = rollDie()
roll
Function Arguments
In the simple function we defined above there were no arguments passed to the function. Arguments can be thought of as variable place holders that the user will pass to the function for there specific need. For example, you could generalize the function above so that it could simulate rolling a die with a user-defined number of sides.
# Define a function to simulate rolling a n-sided die
def rollDie(sides):
value = random.randint(1, sides)
return value
In the code above, you can see that we used an argument "sides" to be a place holder for the number of sides the user would want the die to have. Now if a user wanted to roll a twelve-sided die instead of six-sided die they could do so.
# Run rollDie() for a twelve-sided die
roll = rollDie(sides = 12)
roll
Note that you do not need to include the argument name in the function call, for example the code below would work as well.
# Run rollDie() for a twelve-sided die
roll = rollDie(12)
roll
Default Arguments
Another option you have when defining function arguments is to set a default value. When we defined rollDie() with the sides argument, there was no default value. This means that in order to run the function, the user must pass a value to the variable "sides". Sometimes it can be useful to set a default parameter value when defining a function. In the rollDie() example, we could make sides default to six since we know that six is a very common number of sides for a die to have.
# Define a function to simulate rolling a n-sided die, with a default side value of six
def rollDie(sides = 6):
value = random.randint(1, sides)
return value
Note that in the function defined above, a user could still specify the number of sides they want the die to have by passing in a value for the "sides" argument. But if they do not specify a "sides" argument, the function will still run and default to six sides.
# Run rollDie() for a three-sided die
roll = rollDie(3)
roll
# Now run rollDie() with default number of sides
roll = rollDie()
roll
Example Walk-Throughs with Worksheets
Video 1: Writing Functions in Python
Video 2: Simulating the Birthday Problem
Video 3: Simulating Guessing on an Exam
Practice Questions
Q1: What would run after executing fortuneTeller() given the following function definition with n equal to 2?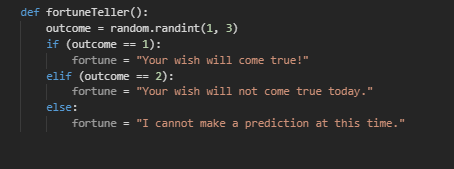
Q2: Which of the following statements is true regarding Python function arguments?
Q3: When writing a function in Python what keyword must the function declaration always begin with?
Q4: What will print after executing this Python code chunk?